Write clean and maintainable code
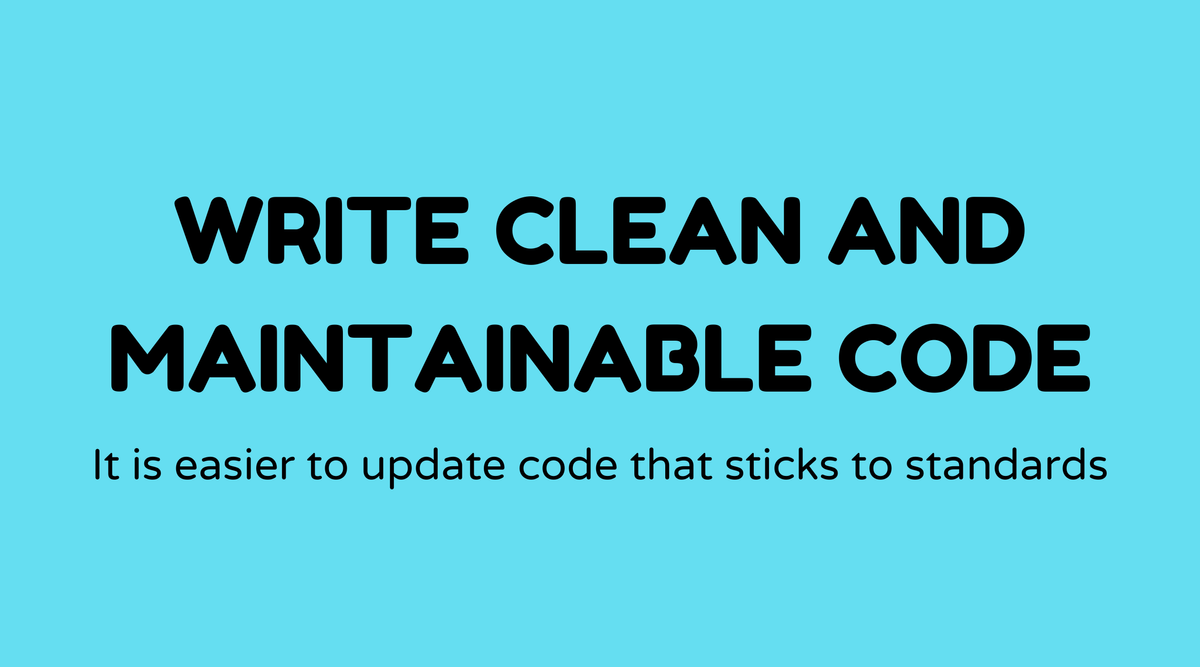
Overview of Writing Clear and Maintainable Code:
In today's fast-changing technology landscape, writing clear and maintainable code is essential for developers. This practice ensures that your projects are scalable and easy to modify, facilitating smooth collaboration with fellow team members. When code is clear, it becomes understandable; when it’s maintainable, it can be adapted easily. As we examine this topic, we will discuss the significance, strategies, and examples of writing clean, maintainable code that endures over time.
Why Writing Clear and Maintainable Code Matters:
Writing clear code is not just a matter of visual appeal—it is a fundamental practice for the long-term success of any project. Clear code is straightforward to read, comprehend, and alter, enabling developers to swiftly troubleshoot issues or enhance functionalities. This improves productivity, diminishes bugs, and simplifies the onboarding of new developers. Furthermore, when code is maintainable, it is easier to revise or update, which is essential for keeping software relevant as technologies and user needs evolve. In software development, time translates to resources; thus, maintaining code preserves both.
What is Clear and Maintainable Code:
Clear and maintainable code is essentially code that is both functional and elegant. It is centered on clarity, simplicity, and efficiency. Key attributes include:
- Readability: The code should be easily comprehensible by others, employing clear naming conventions and minimal complexity.
- Consistent Style: Using a uniform coding style ensures that the entire team’s code appears as if written by a single entity.
- Logical Organization: Code should be structured logically, with clear separations of responsibility (e.g., MVC - Model View Controller pattern).
- Documentation: While code itself should be self-explanatory, comments and documentation can provide additional context and reasoning for complex logic.
- Modularity: Dividing the code into reusable pieces or modules can facilitate maintainability.
How to Write Clear and Maintainable Code:
Creating clear and maintainable code is both an art and a skill. Here’s how to achieve it:
-
Use Descriptive Names: Select variable and function names that indicate their purpose clearly. Avoid ambiguous or abbreviated names.
-
Follow Consistent Naming Conventions: Whether you use camelCase or snake_case, choose a style and use it consistently throughout the codebase.
-
Limit Line Length: Keep line lengths between 80-100 characters for clarity.
-
Comment Wisely: Use comments to explain "why" certain decisions were made, but avoid reiterating "what" the code does, which should be evident.
-
Use Functions for a Single Task: Each function should handle one responsibility, making it easier to test and modify.
-
Refactor Regularly: Continuously improve the code while working on it to keep it flexible and adaptable.
-
Write Tests: Automated tests verify that the code performs as expected and simplify future modifications.
-
Employ Version Control: Utilize tools like Git to track changes and collaborate more effectively.
Examples of Clear and Maintainable Code:
Consider these examples and guidelines:
-
Poor Example:
def calc(a, b): if a == 0: return b else: return a + b
-
Better Example:
def calculate_sum_or_return_second_if_first_is_zero(first_number, second_number): if first_number == 0: return second_number return first_number + second_number
The better example employs descriptive naming, enhances readability, and conveys its purpose more effectively.
FAQs
What tools can help ensure clear code?
There are numerous tools available, such as ESLint for JavaScript and Pylint for Python, which enforce coding standards and style.
How can I encourage a team to write maintainable code?
Adopt a shared style guide, implement code reviews for ongoing feedback, and ensure everyone has a grasp of the importance of code quality.
Is it necessary to write comments if the code is clear?
Comments should clarify the "why" behind certain decisions; however, the "what" should be apparent from the code itself.
Why is modular code preferable?
Modular code allows different program parts to be developed, tested, and debugged separately, simplifying maintenance and enhancing reusability.
How do naming conventions impact code maintainability?
Consistent naming reduces cognitive load and the time needed to understand the codebase, making it more intuitive.
Where can I learn more about coding practices?
Books such as "Clean Code" by Robert C. Martin are excellent resources. Additionally, YouTube tutorials and articles by seasoned software engineers can provide valuable insights.