Codebase with clean and maintanable code
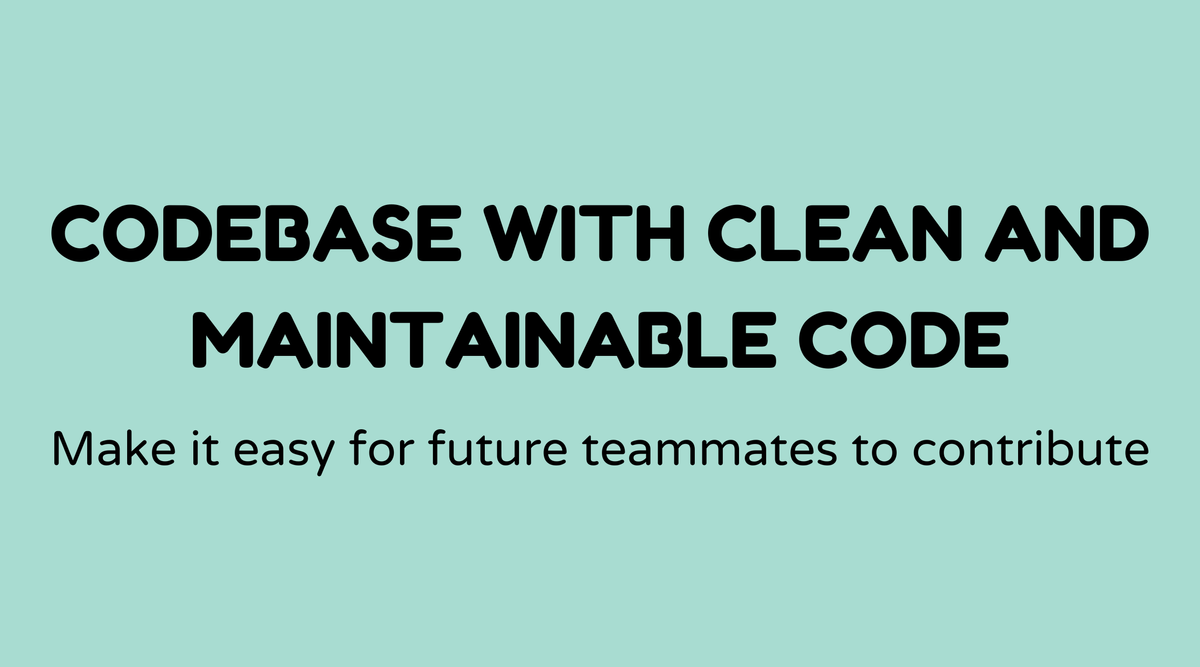
Overview of Codebase with Clear and Manageable Code:
Navigating through a codebase can often feel like traversing a dense forest, where every line of code is a path that leads you closer to or further away from your end goal. A clear and manageable codebase ensures those paths are well-marked, easy to follow, and efficient. It's akin to transforming that dense forest into a beautiful park, where everything is orderly and easy to understand.
In the realm of software development, having a clear and manageable codebase is not just a luxury; it's a necessity. It boosts productivity and decreases the chances of errors while making the code inviting for new developers. But what exactly makes code "clear" and "manageable"? Let's find out.
Why a Codebase with Clear and Manageable Code Matters:
Understanding why clear and manageable code matters can be as refreshing as a morning cup of coffee. It boils down to these beneficial aspects:
-
Ease of Understanding and Onboarding: For anyone new to the team or a specific project, diving into convoluted code is discouraging. A clear codebase makes onboarding seamless, like a gentle river ride.
-
Reduced Bugs and Errors: With clarity comes a lower likelihood of mistakes, similar to how organized spaces reduce the chances of mishaps.
-
Enhanced Collaboration: When everyone can grasp the code, collaboration becomes more harmonious, like a well-orchestrated symphony.
-
Cost and Time Efficiency: Debugging and adding features require much less time, cutting down unnecessary development expenses.
Clear code is not just developer-friendly; it benefits the business as well. A great resource on this topic is the book "Clean Code" by Robert C. Martin, which discusses these benefits in detail. For an even broader understanding, check out this YouTube video on clear code principles.
What is Clear and Manageable Code:
To grasp the essence of clear and manageable code, think of how a well-organized kitchen works. Everything is in its place, labeled clearly, and every process is easy to follow. In coding terms, here are the key features:
-
Readability: Code should be easily understandable by humans, not just computers. This means using clear naming conventions, appropriate comments, and logical flow.
-
Modularity: Like separating ingredients in a kitchen, code should be divided into small, manageable parts (functions, classes).
-
Consistency: It’s like following specific cooking methods – consistency in style and conventions makes the code predictable and trustworthy.
-
Documentation: Every part of your codebase should be accompanied by information about what it's doing and why – like a cookbook’s step-by-step guide.
-
Testing: Ensure everything works as it should with consistent testing.
How to Achieve Clear and Manageable Code:
Creating a codebase that's clear and manageable involves thoughtful steps and practices. It's not something you achieve overnight, but rather a continuous effort.
-
Adopt a Coding Standard: Establish rules for things like naming conventions and file organization. The Python PEP 8 guide is a great starting point for Python developers.
-
Consistent Refactoring: Regularly reviewing code to enhance it without altering its functionality can keep it organized and effective.
-
Comprehensive Testing: Utilize unit tests, integration tests, and code reviews to ensure quality and functionality.
-
Automated Tools: Employ tools like linters and formatters to catch errors early and enforce style guidelines automatically.
-
Code Reviews: Regularly review code with peers, bringing fresh perspectives.
Here are some practical steps detailed in this insightful article on writing maintainable code.
Examples of Clear and Manageable Code:
Examining software companies like Google or Microsoft, their success partly stems from solid coding practices that emphasize clarity and manageability. Open-source projects like React and Django are often praised for their clear code, reflecting a community-wide commitment to clarity and quality.
Here's a simple example to illustrate:
def calculate_area_of_rectangle(length, width):
"""Calculates the area of a rectangle given the length and width."""
if length <= 0 or width <= 0:
raise ValueError("Length and width must be positive numbers.")
return length * width
In this snippet, clear naming conventions and comments make the code self-explanatory, while an error check ensures robustness.
FAQs
- Can existing codebases be made clear and manageable?
- Absolutely! Through refactoring and implementing coding standards, any codebase can be transformed into a more maintainable state.
- Do clear and manageable codes guarantee error-free software?
- While they greatly reduce errors, regular testing and reviews are also essential.
- How often should code refactoring be done?
- It should be an ongoing process, ideally integrated into regular development cycles.
- Is there a universal coding standard?
- No, standards can vary by language and team preference. However, it's vital for a team to adhere to the same set of standards.
- What's the first step in cleaning a messy codebase?
- Start by defining your coding standards and gradually refactor code.
- Are there tools to help maintain a clear codebase?
- Yes, popular tools include linters like ESLint for JavaScript or Flake8 for Python, which help maintain quality.
A clear and manageable codebase is the cornerstone of efficient and error-free software development. By understanding its importance and applying the right practices, you not only improve the software but also enhance the satisfaction and productivity of everyone who works with it.
For more insights, visit the GitHub repository standards to see how they efficiently manage vast codebases.