Code documentation
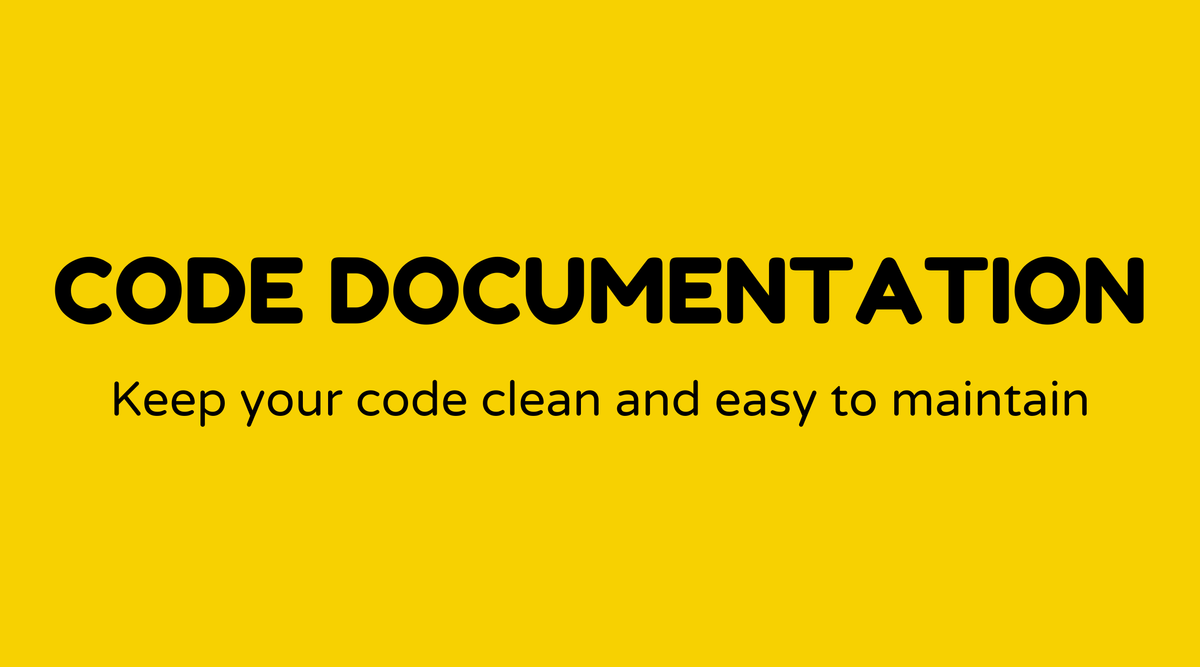
Overview of Code Documentation:
Code documentation plays a vital role in software development. It consists of descriptive notes embedded in your code that explain what the code does, how it operates, and the reasoning behind particular choices. Imagine navigating a new city without a map—quite challenging, right? Code documentation serves as that essential map for anyone interacting with a codebase, including yourself in the future!
Importance of Code Documentation:
Without adequate documentation, code can quickly become a confusing maze within your project, where valuable time is wasted. Documentation enhances readability, making it simpler for others to grasp your logic and workflow. Moreover, it facilitates easier debugging and maintenance. It’s also vital for teamwork; collaboration is hindered when team members lack a clear understanding of each other's contributions. Well-crafted documentation can prevent numerous frustrations later on. According to a study by Atlassian, documentation can boost productivity by 25%!
Understanding Code Documentation:
Code Documentation refers to written text or annotations accompanying software code, detailing its functionality. It can be broadly categorized into internal and external documentation.
- Internal Documentation: This comprises comments embedded within the code itself—those helpful notes using programming hashtags that clarify what the code is doing at specific points.
- External Documentation: This includes comprehensive documents such as README files, user manuals, and software design documents that provide an overview of the system, including installation instructions, system requirements, and user guidelines.
How to Create Code Documentation:
Crafting code documentation goes beyond simply writing a few lines. It requires a thoughtful approach:
-
Identify Purpose: Before writing, consider the function or module’s intent. What is it designed to accomplish? Start with a summary.
-
Inline Comments: Use comments in the code to clarify complex logic. These should make things clearer rather than more confusing. For example:
# Calculate the factorial of a number
-
Descriptive Naming: Choose clear, descriptive names for variables and functions. This serves as a subtle form of documentation.
-
Utilize Docstrings: Languages like Python rely on docstrings. These are multi-line comments describing a module, class, or function.
def add(a, b): """Return the sum of two numbers a and b.""" return a + b
-
Develop External Documents: For larger projects, document the architecture, API references, and user manuals. Tools like Javadoc, Doxygen, or Sphinx can assist in automating aspects of this process.
-
Regular Updates: Incorporate updates to documentation into your development routine whenever the code changes. Version control can help track these updates.
Examples of Code Documentation:
Here are some notable examples of repositories with excellent documentation:
-
TensorFlow: An open-source library featuring comprehensive API documentation and usage guides. Explore their GitHub repository for a thorough overview.
-
React: A widely-used JavaScript library for building user interfaces, showcasing a great example of effective documentation.
These examples illustrate how thorough documentation substantially enhances usability and developer experience within a project.
FAQs
1. How frequently should code documentation be updated?
- It should be updated as often as the code changes! This should be an ongoing practice to maintain accuracy and relevance.
2. What tools are available for code documentation?
- Common tools include Sphinx, Doxygen, and Javadoc, which help automate documentation processes.
3. Should documentation be in a separate file or within the code?
- A combination of both is optimal. Inline comments can clarify complex logic, while separate documents can serve as overall guides and reference materials.
4. Which is more crucial: comprehensive or concise documentation?
- Striking a balance is essential. Be concise, but do not overlook key details that aid in comprehension.
5. Can documentation improve my coding skills?
- Definitely! Documenting your code requires a deep understanding, which can lead to better, more thoughtful coding practices.
6. What is the best way to start documenting an existing project?
- Begin with high-level overviews and progressively address detailed aspects of the project.